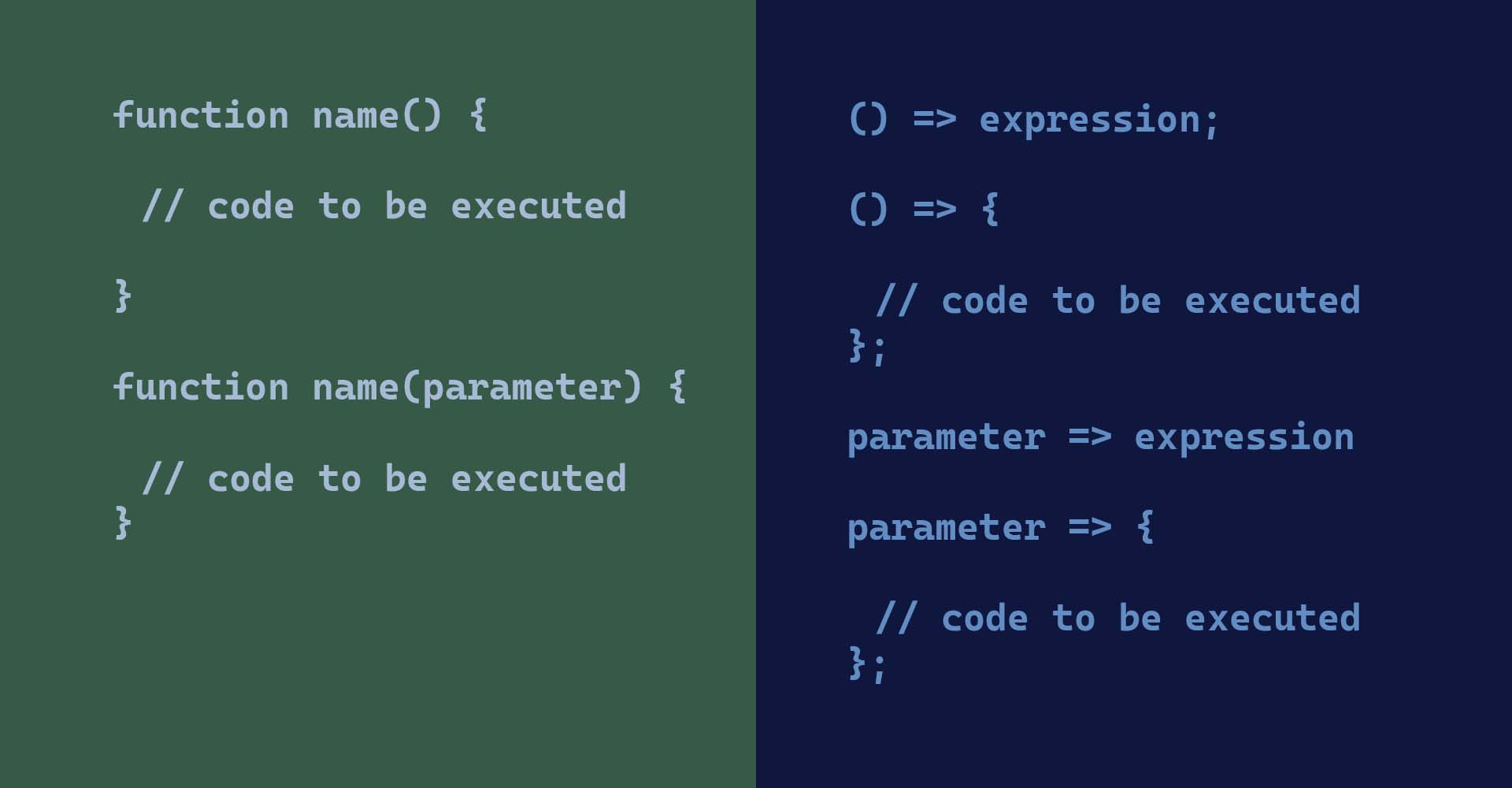
Arrow Functions vs Regular Functions in JavaScript
This tutorial shows the primary differences between the newly introduced arrow functions in ES6 vs the existing regular functions in JavaScript using the practical examples.
This tutorial shows the differences between the newly introduced arrow functions in ES6 vs the existing regular functions in JavaScript using the practical examples.
Syntax
The arrow functions can be written without the function keyword. We can also omit the paranthesis in case of only one argument as shown below.
Regular Function
The regular function starts with the keyword function followed by the parameters within the paranthesis followed by the function body within the curly braces.
// Regular Function - Without arguments, Without return, returns undefined
function myFunctionName() {
// code to be executed
}
// Regular Function - With arguments, Without return, returns undefined
function myFunctionName(parameter1, parameter2, parameter3) {
// code to be executed }
// Regular Function - With arguments, With return
function myFunctionName(parameter1, parameter2, parameter3) {
// code to be executed
return <variable or value>;
}
The function parameters can be zero to n depending on the function declaration. It may or may not return. The arguments or the parameter values received by the function behave as local variables.
Arrow Function
The arrow functions are introduced in ES6 and allows us to write shorter function syntax.
// Without arguments
() => expression
() => {
// code to be executed
}
// Single argument
parameter => expression parameter => {
// code to be executed
}
// Multiple arguments
(parameter1, parameterN) => expression (parameter1, parameterN) => { // code to be executed }
The arrow functions can be async as shown below.
async parameter => expression async parameter => { // code to be executed } async (parameter1, parameterN) => expression async (parameter1, parameterN) => { // code to be executed }
Semantic Differences
The arrow functions are compact or concise as compared to the regular functions in terms of syntax as mentioned in the previous section. There are few semantic differences between both the types as listed below.
- The arrow functions introduced in ES6 don't have their own bindings to this, arguments, and super keywords. In the case of browsers, the arguments object remains undefined and the this keyword references to the default Window object, whereas we can't use the keyword super within the arrow functions.
- We cannot use the arrow functions as constructors, hence we cannot call them using the new keyword like we do with regular functions.
- We cannot use yield within the body of arrow functions and cannot create them as generator functions.
- An explicit return is required in case of arrow functions using the block body using curly braces. In case return is not used, the functions returns undefined similar to a regular function.
- An arrow function cannot contain a line break between the paranthesis and arrow, though line break after the arrow is allowed.
Examples
This sections shows the differences between the arrow functions and regular functions by examples.
Regular Function
The regular functions can be assigned to a variable as shown below. We can assign a different function to the variable at runtime to override the existing function.
// Regular function without assignment function add(num1, num2) { return num1 + num2; } // Regular function, assigned to a variable let addVar = function add(num1, num2) { return num1 + num2; }; // Calling regular function let num1 = add(5, 10); let num2 = addVar(5, 10); // Console logs console.log(`Num1: ${num1}`); console.log(`\nNum2: ${num2}`);
// Output
Num1: 15 Num2: 15
In the above example, both the console logs print 15 as the result of calling the add and addVar functions.
Arrow Functions
This sections shows the examples of arrow functions using both expression and function body.
// Arrow function using expression
let add10 = num => num +10; let add1 = (num1, num2) => num1 + num2; let comp1 = (num1, num2) => num1 > num2 ? "Num1 is greater than Num2." : "Num2 is greater than Num1."; // Arrow function using curly braces let add2 = (num1, num2) => { return num1 + num2; }; let comp2 = (num1, num2) => { return num1 > num2 ? "Num1 is greater than Num2." : "Num2 is greater than Num1."; }; // Calling arrow function
let add10Result = add10(5); let addResult1 = add1(5, 10); let addResult2 = add2(5, 10); let compResult1 = comp1(5, 10); let compResult2 = comp2(15, 10); // Console logs
console.log(`Add 10 Result: ${add10Result}`);
console.log(`\nAdd Result 1: ${addResult1}`);
console.log(`\nAdd Result 2: ${addResult2}`);
console.log(`\nComparison Result 1: ${compResult1}`);
console.log(`\nComparison Result 2: ${compResult2}`);
// Output
Add 10 Result: 15
Add Result 1: 15
Add Result 2: 15
Comparison Result 1: Num2 is greater than Num1.
Comparison Result 2: Num1 is greater than Num2.
Anonymous Functions
We can replace the traditional way of writing the anonymous functions using the arrow functions as shown below. The arrow functions makes it a lot simpler to write anonymous functions in JavaScript.
// Traditional anonymous function without any name let anonymous1 = (function (num1, num2) { return num1 + num2; }); // Arrow function without any name
let anonymous2 = (num1, num2) => { return num1 + num2; }; let anonymous1Result = anonymous1(5, 10); let anonymous2Result = anonymous2(5, 10); // Console logs console.log(`Anonymous 1 result: ${anonymous1Result}`); console.log(`\nAnonymous 2 result: ${anonymous2Result}`);
// Output
Anonymous 1 result: 15 Anonymous 2 result: 15
Summary
This tutorial explained the differences between the regular functions and the arrow functions in JavaScript. It explained the differences in terms of syntax and coding.